# Unit 1 - Primitive Types
## 1.1 Why Programming Why Java
- [ ] Learn about the Java™ programming language and create your first Java program.
- [ ] I will be able to write a basic java program
- [ ] Learn some basic rules of Java programming by identifying and correcting errors in code.
- [ ] I will be able to indentify basic syntax of a java program
- [ ] Generate outputs to a console by calling System class methods.
- [ ] Understand the difference between the `print()` method and the `println()` method.
Java is an Object Oriented Program. Within every Java program begins with the creation of a class. Consider a class as a blueprint for your program. In this instance, we created a class called *MyFirstClass*. Within the class there is a main method that is required to execute the program. Below is a simple program that will print "Hello, World".
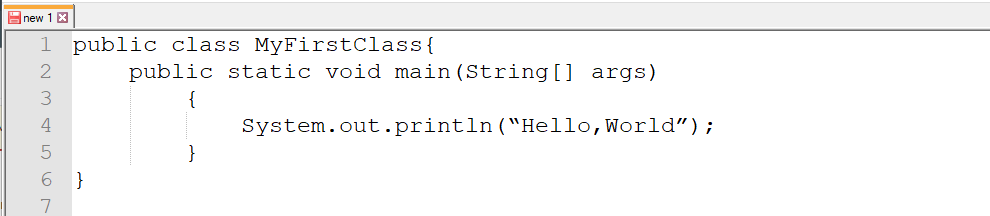
Notice that with every { , there is a corresponding } . You may hear me refer to it as a curly brace. A missing a curly brace is one of the most common errors among new programmers. The name 'public' allows users to be able to see the specific parts of your program. Notice also that the name of the class starts with a **capital letter**. The main class will always start with a capital letter.
In Java there is a "main method" that is required for all java programs. The code will always be the same. It looks like:
```java
public static void main(String args[])
```
**`public`** is an access specifier. As you may infer, public is public and everyone will have access to it.
**`static`** is a keyword in java. A `static` method belongs to the class and not to the object (We will learn more about this later!). A `static` method can **only** access static data. It is a method which belongs to the class and not to the instaniation of the object. There are differences between `static` nested class and `non-static` nested class.
| | Static method | Non-static method |
| ----- | ----- | ----- |
| Definition | A static method is a method that belongs to a class, but it does not belong to an instance of that class and this method can be called without the instance or object of that class. | Every method in Java defaults to a non-static method without a static keyword preceding it. non-static methods can access any static method and static variable also, without using the object of the class. |
| Accessing members and methods | In the static method, the method can only access only static data members and static methods of another class or the same class but cannot access non-static methods and variables. | In the non-static method, the method can access static data members and static methods as well as non-static members and methods of another class or the same class. |
| Binding process | The static method uses compile-time or early binding. | The non-static method uses runtime or dynamic binding. |
| Overriding | The static method cannot be overridden because of early binding. | The non-static method can be overridden because of runtime binding. |
| Memory allocation| In the static method, less memory is used for execution because memory allocation happens only once because the static keyword fixed a particular memory for that method in ram. | In the non-static method, much memory is used for execution because here memory allocation happens when the method is invoked and the memory is allocated every time when the method is called. |
**`void`** is also a keyword. It is a 'return type'. What does `void` return? Nothing! It is used to specify that a method **does not** return anything. If the main method is not void, it will return an error.
**`main`** is the name of the Java main method. It identifies the starting point of a Java program. If we change the name while initiating the main method, it will return an error.
**`String[] args`** It is an array of string type. It stores Java command-line arguments and is an array of type `java.lang.String` class. The name of the String array is `args`. It is not fixed and the user can use any name in place of it (It is not recommended as some IDEs will require args in the main method.).
Notice that within the main method there is a print statement. Let's break down this print statement.
System.out.println("Hello, World");
- [ ] System is a class defined in the java lang package.
- [ ] out is a public static field of the PrintStream type.
- [ ] println() is invoked by the PrintStream class.
- [ ] NOTE println() when executed will return to the next line. Whereas print() will execute the string literal but not return to the next line. You can also create a new line inside a print statement by adding \n anywhere inside the quotation marks of a print statement.
-------------------------------------------------------------------------------------------------------------------
Take a look at the diagram below:

What observations can you make about the diagram?
A powerful tool that you can use in OOP is the concept of `inheritance`. `Inheritance` is the concept where a Java Class can inherit properties from another class. You can think of this as a child inheritiing characteristics of a parent. Java refers to this as **extending** a class. The Class that is being extended or "inherited" is called a **superclass**. The class that extends or "inherits" is referred to as a **subclass**. We will explore this in more detail in Chapter 4.
----------------------------------------------------------------------------
## 2.1 Instances of Classes
- [ ] Explain the relationship between a class and an object
- [ ] I will be able to create a poster demonstrating classes and objects
As stated earlier, a *class* is a blueprint of an object. So we can also say that an *object* is an instance of a class. What we define as a class determines what objects that will be associated with the class and how things will operate within it. For example, we can define `human` as a class. We can assign attributes to that class like a nose, ears, eyes, hands, feet, etc. **NOTE:** Attribute and state are interchangable terms.
We can also create a *method* that will call the attributes within a class. For example, we can create a method called running or sleeping. Now each of these methods will use an attribute or attributes of its class to perform something within the `human` class. If we create an instance of the `human` class, let say Object John , then John will have all the attributes (nose , ears, eyes, hands, legs) unique to it and will also have access to these methods to carry out basic functions. **NOTE:** Method and behavior are interchangable terms.
The value of the attributes define the state of the object. What makes this efficient is that the class does not hold any space in the memory because we just create a definition. The moment we *instantiate* the class the object will require a dedicated space in memory. Thus we can say that a Class defines how the object should look and object is the actual representation of the same.
-------------------------------------------------------------------------------------------
**Mr Potato Head Activity:**
Supplies will be provide for you to complete this activity.
Split into pairs.
Each pair will create:
a potato head character.
a list describing what it is, what characteristics it has, and what it can do(actions).
write PROPERTIES/ characteristics
write METHODS/ actions
What are the object oriented concepts to your Mr. Potato Head?
Class:
Object:
Properties:
Methods:
Visually demonstrate the properties of inheritance by creating a subclass(es) of your Superclass.
Include the Characteristics and actions of the subclass(es).
-------------------------------------------------------------------------------------------
**Example:**
```java
class Bicycle {
// attribute or state
int gear = 1;
String color = white;
// behavior or method
public void pedaling() {
System.out.println("Pedaling to accelerate!");
}
}
```
**Sample Output**
```java
My bicycle has 1 gear and is painted white!
Pedaling to accelerate!
```
Sample Solution
```java
class Main {
public static void main(String[] args) {
Bicycle myBicycle = new Bicycle(); // Creation of a constructor
// We will learn more about this later
myBicycle.pedaling(); //Calling Method
}
}
public class Bicycle { //Instaniation of the constructor created in the Main Class
private int gear;
private String color;
// Constructor to initialize gear and color
public Bicycle() {
gear = 1;
color = "white";
}
// Method to pedal the bicycle
public void pedaling() {
System.out.println("My bicycle has " + gear + " gear and is painted " + color + "!");
System.out.println("Pedaling to accelerate!");
}
}
```
------------------------------------------------------------------------------------------------------------
**Things to Remember**
Object-oriented Programming Facts:
- [ ] Properties are the characteristics of your objects
- [ ] Object-Oriented programming focuses on the data represented in the problem
- [ ] Object-Oriented programs remove details from many programs, making the code rewrite less frequent.
- [ ] Methods are the actions that your object can take.
- [ ] A class is like a blueprint or a recipe.
- [ ] A class is used to create an instance of a class, called an object
----------------------------------------------------------------------------
### 5.3 Documentation with Comments
- [ ] Understand how to properly comment code.
- [ ] Implement precondition and postcondition commenting to summarize methods.
- [ ] Use single-line comments to make code more readable and understand what tasks it performs.
- [ ] I will practice industry standard commenting standards in my programs.
Typing comments within your program is considered a professional practice, and I expect you to comment your code as appropriate throughtout the year. When other people read your program, they may not understand the purpose of the variables and/ or methods in
your program. That's why it's important to document your code with comments. A comment is a piece of text that the
computer will not recognize as code and will not execute. It's only there for the other programmers to read. While you can write whatever
you want inside a comment, you should stick to explaining what your code does to document it properly.
There are three ways in Java to create a comment:
// Using these two slashes will create a single-line comment.
/* Using the slash and an asterisk will create a multi-line comment for longer explanations. Be sure to close it with an asterisk, then a slash. */
/** Using the slash and two asterisks will create a Java API documentation comment. In these comments, you can use tags to specify the parameters of a method and the return values of the method. These comments aren't as important for the AP CSA curriculum. **/
@param -- explanation of parameter
@return -- explanation of what the method returns
Example of appropriate comments with a program:
With **every** program that you submit, I want you to list the following:
- your name/ partners name (if applicable)
- the date of submission
- Period
- and the assignment
Please follow the convention shown below.
```
/*
* Programmer(s):
* Date:
* Section: Period #
* Assignment:
*/
```
### Assignment 1.1.1
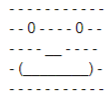
Create the happy face image above using the following criteria:
- [ ] Create a class
- [ ] Create a main method
- [ ] Use System.out.print at least two lines
- [ ] Use System.out.println at least three times
Sample Solution
```java
public class Main
{
public static void main(String[] args)
{
System.out.println("----------");
System.out.print("--0----0--\n");
System.out.println("----__----");
System.out.print("-(______)-\n");
System.out.println("----------");
}
}
```
### Assignment 1.1.2
**Personal Timeline**
For this assignment, you will create a program that outputs a personal timeline of events in your life. The timeline will proceed vertically. Earlier events will be displayed nearer the top of the timeline while later events are displayed near the bottom.
Include seven meaningful events in your life. No repetitive events such as "started kindergarten, started first grade, etc".
Make it look professional. HINT: use \t
More examples of Escape Sequences can be found [here](https://ap-csa-java.github.io/CSA_JAVA-Course/Chapter_1/Unit2.html#string-objects-concatenation-literals-and-other-things).
```
Year Age Description
1971 0 years old Born in Long Beach
1976 5 years old Started kindergarten
1989 17 years old Graduated high school
1995 24 years old Graduated college Long Beach State
1998 27 years old Got Married
1998 27 years old Began teaching career
2021 50 years old First year at CAMS
```
Sample Solution
```java
class Main {
public static void main(String[] args) {
System.out.println("Year \t\t Age \t\t\t Description");
System.out.println("1971 \t 0 years old \t\t Born in Long Beach");
System.out.println("1976 \t 5 years old \t\t Started Kindergarten");
System.out.println("1989 \t 17 years old \t\t Graduated high school");
System.out.println("1995 \t 24 years old \t\t Graduated college Long Beach State");
System.out.println("1998 \t 27 years old \t\t Got Married");
System.out.println("1998 \t 27 years old \t\t Began teaching career");
System.out.println("2021 \t 50 years old \t\t First year at CAMS");
}
}
```
## 1.2 Variables and Data Types
- [ ] Learn to use variables so that the same algorithm runs using different input values.
- [ ] Determine the appropriate use for specific variables and data types.
- [ ] I will be able to write a program using primitive and non-primitive types.
There are eight primitive types in Java. In no particular order, they are int, double, boolean, float, char, byte, long and short. For this course we will be using only int, double, and boolean.
- [ ] **int** is short for integer deals with whole numbers and uses 32 bits of memory
- [ ] **double** is a 64 bit data type that stores decimal numbers
- [ ] **boolean** is used to represent true or false values
- [ ] **float** is very similar to a double but uses less memory
- [ ] **char** is a 16 bit unicode with a minimum value of \u0000 (0) to uffff (65,535)
- [ ] **byte** is an 8 bit integer with a range from -128 to 127
- [ ] **long** is a 64 bit datatype with a minimum value of -2^63 to 2^63
- [ ] **short** is a 16 bit datatype with a minimum value of -32,768 to 32,767
Non-primitive data types include a String, Arrays and Classes.
- [ ] **String** is a data type that is a collection of characters encompassed by quotes
- [ ] **Arrays** are used to store multiple values (think 'list')
- [ ] **Class** is an object constructor, blueprint, for creating objects.
**Variable Naming Convention**
- [ ] Always choose a name that indicates the purpose of the variable
- [ ] A variable cannot begin with a number
- [ ] A variable must not have spaces
- [ ] A variable cannot begin with any special characters
- [ ] A variable cannot have the same name as a Java keyword
When you declare a variable, you must state the variable type along with the variable name followed by the value assignment to the variable. For example: *int height = 72;*
Once you have declared the variable type, you do not need to do it again to reassign a value to the variable. Example: *int height = 72;height = 70;*
If you declare a variable as *final* you cannot change the value of it. We can refer to the *final* variable as immutable (unchanging). Another variable norm is that *final* variables are written as ALL CAPS to remind you that they cannot change. Example: final double PI = 3.14159;
### Assignment 1.2.1
**Allowance**
Write a program that will determine who receives an allowance or not. Use the information below as a guide:
- [ ] Allowance is always $30.00/ week
- [ ] Non-teenagers do not receive an allowance
- [ ] Jon is 16 years old
- [ ] Ed is 12 years old
- [ ] Michael was born fours years before Jon
- [ ] Print out who receives an allowance and who does not by using an int, double and boolean variables.
```java
int JonsAge = 16;
boolean Teenager = true;
double allowance = 30.00;
System.out.println("Jon's age is " + JonsAge);
System.out.println("Eligible for allowance? " + Teenager);
System.out.println("Jon's weekly allownace is " + allowance);
```
**Example Output:**
Jon's age is 16
Eligible for allowance? True
Jon's weekly allowance is $30.00
Ed's age is 12
Eligible for allowance? False
Ed's weekly allowance is $0.00
Michael's age is 20
Eligible for allowance? False
Michael's weekly allowance is $0.00
### Assignment 1.2.2
Use a Scanner object to create an input feature in your program. Click on [Scanner_Input](https://github.com/AP-CSA-JAVA/CSA_JAVA-Course/files/8455326/Scanner_Input.pptx) to learn more. An example of a Scanner Input can be found [here](https://replit.com/@jonvirak/JavaScannerBasics#Main.java). Create four questions that will ask the user for a string, int, double, and a boolean. From the user's response, you will return (print statement) a sentence that includes the data types listed above.
- [ ] string
- [ ] int
- [ ] double
- [ ] boolean
- [ ] print statement that includes all for data types
--------------------------------------------------------------------------------------------------
## 1.3 Expressions and Assignment Statements
- [ ] Describe the functionality of a program using a single-line comment.
- [ ] Use operators to construct compound expressions.
- [ ] Evaluate the results of an expression with an assignment statement.
- [ ] I will understand and practice proper commenting in programming
Math Operators are a very important part of computing. Below are the most common operators that we will be using in this course.
| Symbol | Name | Usage |
| :---: | :---: | :---: |
| ( ) | Parentheses | You will evaluate based on what is within the parentheses |
| * | Multiplication | multiply two values together |
| / | Division | divide two values |
| % | Modulo | the remainder after dividing two values |
| + | Addition | add two values together |
| - | Subtraction | subtract two values |
**Note** When you divide an integer by zero your program will return an ArthmeticException error.
**Please Excuse My Dear Aunt Sally**
In Java, the order of operation works pretty much the same with the exception of the modulo.Take for example the following math equation: **5 + 12 * 18 - 10 / 2 % 2**
| Step | Operation | Result |
| :---: | :---: | :---: |
| 1 | Multiply 12 * 18 | = 216 |
| 2 | Divide 10 / 2 | = 5 |
| 3 | Modulo 5 % 2 | = 1 |
| 4 | Add 5 + 216 | = 221 |
| 5 | Subtract 221 - 1 | = 220 |
JAVA Order of Operations
| Level | Operator | Description | Associativity |
| :---: | :------: | :---------: | :-----------: |
| 16 | (), [], new, . , :: | parentheses, array access, object creation, member access, method reference | left-to-right |
| 15 | ++, -- | unary post-increment, unary post-decrement | left-to-right |
| 14 | +, -, !, ~, ++, -- | unary plus, unary minus, unary logical NOT, unary bitwise NOT, unary pre-increment, unary pre-decrement | right-to-left |
| 13 | () | cast | right-to-left |
| 12 | * / % | multiplicative | left-to-right |
| 11 | + - , + | additive, string concatenation | left-to-right |
| 10 | <<, >>, >>> | shift | left-to-right |
| 9 | <, <=, >, >=, instanceof | relational | left-to-right |
| 8 | ==, != | equality | left-to-right |
| 7 | & | bitwise AND | left-to-right |
| 6 | ^ | bitwise XOR | left-to-right |
| 5 | \| | bitwise OR | left-to-right |
| 4 | && | logical AND |left-to-right |
| 3 | \|\| | logical OR | left-to-right |
| 2 | ?: | ternary | right-to-left |
| 1 | =, +=, -=, *=, /=, %=, &=, ^=, \|=, <<=, >>=, >>>= | assignment | right-to-left |
| 0 | -> | lambda expression, switch expression | right-to-left |
-----------------------------------------------------------------------------------
### Assignment 1.3.1
**Calculate your weight on Jupiter**
```
The expression F=ma allows you to calculate your weight (in newtons) based on your mass
(in kilograms) the acceleration due to gravity on Earth (9.81 m/sec2). A typical mass of a
human might be 58.9 kg.
F = ma
F = (58.9 kg)(9.81 m/s^2)
F = 578 Newtons
One newton is equal to 0.225 lbs. So on Earth, this human weighs 130 lbs.
F = (578 N)(0.225 lbs/ 1 N) = 130 lbs
But Jupiter is more massive than the Earth. The force of gravity is stronger, making the
acceleration due to gravity much larger (24.79 m/sec^2). Below is a list of variables that
will be used in the program.
Using the equation below, you can determine what your weight on Jupiter would be in pounds
and not have to worry about converting units.
(weightOnJupiter) = (weightOnEarth)/1 x (jupiterGravity)/(earthGravity)
weightOnJupiter = 130
earthGravity = 9.81
jupiterGravity = 24.79
**weightOnJupiter = weightOnJupiter / earthGravity * jupiterGravity**
```
**Using the chart below, create a program called GalaxyWeight that outputs information of the user's weight
on four different planets and takes the average of those weights. You will use the scanner class for
this assignment.**
| Planet | Acceleration Due to Gravity (m/sec^2) |
| :---: | :---: |
| Earth | 9.81 |
| Mercury | 3.59 |
| Venus | 8.87 |
| Mars | 3.711 |
| Jupiter | 24.79 |
| Saturn | 11.08 |
| Uranus | 10.67 |
| Neptune | 11.15 |
Sample Solution
```java
import java.util.Scanner;
class Main
{
public static void main(String[] args)
{
int weightOnEarth;
double earthGravity = 9.81;
double mercuryGravity = 3.59;
double venusGravity = 8.87;
double marsGravity = 3.711;
System.out.println("Enter your weight in pounds:");
Scanner sc = new Scanner(System.in);
weightOnEarth = Integer.parseInt(sc.nextLine());
double weightOnMercury = weightOnEarth * mercuryGravity / earthGravity;
double weightOnVenus = weightOnEarth * venusGravity / earthGravity;
double weightOnMars = weightOnEarth * marsGravity / earthGravity;
double weightOnInnerPlanets = (weightOnEarth + weightOnVenus + weightOnMars + weightOnMercury) / 4;
System.out.print("Your weight on Mercury is ");
System.out.println(weightOnMercury + " lbs.");
System.out.print("Your weight on Venus is ");
System.out.println(weightOnVenus + " lbs.");
System.out.print("Your weight on Mars is ");
System.out.println(weightOnMars + " lbs. \n");
System.out.println("Your average weight for the Inner Planets is " + weightOnInnerPlanets + "lbs.");
}
}
**Sample Output:**
Enter your weight in pounds:
150
Your weight on Mercury is 54.89296636085626 lbs.
Your weight on Venus is 135.62691131498468 lbs.
Your weight on Mars is 56.74311926605504 lbs.
Your average weight for the Inner Planets is 99.31574923547399lbs.
```
### Assignment 1.3.2
**Simple Math**
- [ ] Create a program that will ask the user for two distinct numbers (integers)
- [ ] Use the scanner class to receive input from the user
- [ ] Store those values and perform some basic operations with those numbers
**Example Output**
```java
Enter first number:
20
Enter second number:
5
Sum: 25
Difference: 15
Product: 100
Dividing: 4 with remainder 0
```
Sample Solution
```java
import java.util.Scanner;
class Main
{
public static void main(String[] args)
{
// declare two integers a and b
int a;
int b;
// asks the user for the integers and stores it in a and b
System.out.println("Enter the first integer:");
Scanner sc = new Scanner(System.in);
a = sc.nextInt();
System.out.println("Enter the second integer:");
b = sc.nextInt();
System.out.println("When you add the two numbers, the sum is " + (a + b));
System.out.println("When you subtract the two numbers, the difference is " + (a - b));
System.out.println("When you multiply the two numbers, the product is " + (a * b));
System.out.print("When you divide the two numbers, the answer is " + (a / b));
System.out.println(" with a remainder " + (a%b));
}
}
```
----------------------------------------------------------------------------------------------
## 1.4 Compound Assignment Operators
- [ ] Use the compound assignment and increment/decrement operators in a program.
- [ ] I will be able to increment a variable based on java protocol
- [ ] Describe the behavior of a given segment of program code, including the result it produces.
- [ ] I will be able to implement appropriate order of operation as it relates to java
In Java, you can modify a variable by referencing it, performing an operation on it, and assigning the result back to the variable. Thus, you can reference a variable to change its own value. For example, if x equals 0 , the following statement modifies x.
```java
// Will this program run as written? Why or why not?
public class Main
{
public static void main(String[] args)
{
x = 0;
x = x + 1; //common programming convention
System.out.println("x = " + x);
}
}
Output:
x = 1
```
```java
// Compound assignment operators
class Main {
public static void main(String args[])
{
int b = 120; //initial value
b += 10;
int b1 = 120; //initial value
b1 *= 10;
int b2 = 330; //initial value
b2 -= 30;
int b3 = 127; //initial value
b3 %= 7;
System.out.println(b);
System.out.println(b1);
System.out.println(b2);
System.out.println(b3);
}
}
```
**Sample Output**
```java
130
1200
300
1
```
In algebra, this is an impossible statement, but in programming, the assignment operator makes this a simple and logical statement.
To interpret assignment statements such as this, begin with the first instruction to the right of the assignment operator.
In Java, a strong convention is to declare variables close to where they are first used, at the beginning of a code block.
A code block is denoted by curly braces, { }. Notice that the print statement uses quotes around 'x = ' followed by plus(+) sign.
This is referred to as a **concatenation operator** where you can add a string literal to a variable.
Java like other programs has simplified the compound operators. **Compound Assignment Operators** performs two tasks in one step. It performs a mathematical calculation by and assignment. It is written as:
Java supports 11 compound-assignment operators, but for this course we will use just five.
```java
+= assigns the result of the addition.
-= assigns the result of the subtraction.
*= assigns the result of the multiplication
/= assigns the result of the division.
%= assigns the remainder of the division.
```
**Increment and Decrement Operator**
In Java programming, the increment operator (*++*) increases the value of a variable by 1. Similarly, the decrement operator (*--*) decreases the value of a variable by 1. These may also be referred to as prefix and postfix operators.
```java
public class MyClass {
public static void main(String args[]) {
int a = 5;
int b = 5;
int c = 5;
int d = 5;
System.out.println("a = " + ++a);
System.out.println("b = " + b++);
System.out.println("c = " + --c);
System.out.println("d = " + d--);
}
}
```
**Sample Output**
```java
a = 6
b = 5
c = 4
d = 5
```
There is an important difference when these two operators are used as a prefix and a postfix.
- If you use the ++ operator as a prefix like: ++a, the value of a is incremented by 1; then it
returns the value.
- If you use the ++ operator as a postfix like: b++, the original value of var is returned first;
then b is incremented by 1.
### Assignment 1.4.1
**Compound Operators**
```java
public class CompoundOperators
{
public static void main(String[] args)
{
int numPeople = 0;
double totalYears = 0;
double years = 11.5; // I will soon be halfway through my junior year.
totalYears = totalYears + years;
numPeople = numPeople + 1;
}
}
```
- [ ] Rewrite the statements of the program above to use the compound assignment and/or the increment/decrement operators.
- [ ] TODO 1) Add at least three other people to your program, such as siblings, friends, or neighbors.
- [ ] TODO 2) Use people that are in different grades to validate your program can work for different school grades.
- [ ] TODO 3) For their years in school, use values that indicate they will soon be halfway through their current school year, as shown in the provided code.
- [ ] TODO 4)The average years you and your friends, siblings, neighbors have been in school.
- [ ] TODO 5) The total days you have all been in school, assuming you spend 180 days per year in school.
- [ ] TODO 6) The average days you have all spent in school.
```
Sample Output:
I have 11.5 years in school and 0.5 years to graduate.
Total people: 1, total years: 11.5
My best friend has 10.5 years in school and 1.5 years to graduate.
Total people: 2, total years: 22.0
My sister has 8.5 years in school and 3.5 years to graduate.
Total people: 3, total years: 30.5
My neighbor has 5.5 years in school and 6.5 years to graduate.
Total people: 4, total years: 36.0
Average years in school: 9.0
Total days in school: 6480.0
Average days per person: 1620.0
```
----------------------------------------------------------------------------
### Assignment 1.4.2
**Average Test Score**
Directions: Write two programs that will do the following:
**Average Test Score**
- [ ] ask the user for four test scores
- [ ] calculate and show the result
**Cashier Totals**
- [ ] ask the user for the number of burgers sold and how much each one costs
- [ ] ask the user for the number of fries sold and how much each costs
- [ ] display the total items sold
- [ ] display the total sales
**Sample Output:**
```
Enter the first test score:
95
Enter the second test score:
87
Enter the third test score:
74
Enter the forth test score:
75
Average test score: 82.75%
---------------------------------
Enter the number of burgers ordered:
10
Price of a burger:
5.65
Enter the number of fries ordered:
4
Price of fries:
1.95
Total Items Sold: 14
Total Sales: $64.3
```
## 1.5 Casting and Range of Variables
- [ ] Evaluate arithmetic expressions that use manual and automatic casting.
- [ ] Perform mathematical rounding.
- [ ] I will be able to explain why a code segment will not compile or work as intended.
There are some unique features to Java that help programmers create programs that are flexible in how they display data.
We learned earlier that we need to declare a variable by it's type. It can be an int or a double. As you may remember,
an int is any whole negative or positive number.
A double is any number with a decimal. 1.0 is a whole number, but it has a decimal. So, Java considers 1.0 as a double.
We can convert the double by declaring a new variable that changes the double to an int.
```java
double temp = 98.6;
int newTemp = (int)temp;
```
What is the value of newTemp? Did you guess 99? The actual value of newTemp is 98. The variable is not **rounded** it is *truncated*. Java does not round unless you tell it to round.
You will need to be familiar with some terms:
- [ ] **widening** - converting from a smaller data type (int) to a larger data type (double).
- [ ] `byte` -> `short` -> `char` -> `int` -> `long` -> `float` -> `double`
- [ ] **narrowing** - converting a larger data type (double) to a smaller data type (int).
- [ ] `double` -> `float` -> `long` -> `int` -> `char` -> `short` -> `byte`
In this instance, we 'narrowed' the value of temp. There is another term that you need to be familiar with and it is called **casting**. Casting is converting from one data type to another, such as from a *double* to an *int*, potentially losing information.
Take a look at this program:
```java
int eggs = 9;
int dozen = 12;// the variable dozen will not change
System.out.println("Total eggs = " + eggs/ dozen);
Example output:
Total eggs = 0
```
We know that we don't have 0 eggs. We can rewrite our program to show how many eggs we have in decimal form.
```java
int eggs = 9;
int dozen = 12;// the variable dozen will not change
System.out.println("Total eggs = " + eggs/ (double) dozen);
Sample output:
Total eggs = 0.75
```
If you were to print the variables `eggs` and `dozen`, you would see that the values are 9 & 12 respectively.
### Assignment 1.5.1
You will explore the PlanetTravel program called FivePlanetTravel. The program is set up to plan a five-planet tour!:
```
/*
* Activity 1.1.5
*/
public class FivePlanetTravel
{
public static void main(String[] args)
{
// theplanets.org average distance from earth to the planets
int mercury = 56974146;
int venus = 25724767;
int mars = 48678219;
int jupiter = 390674710;
int saturn = 792248270;
// number of planets to visit
int numPlanets = 5;
// speed of light and our speed
int lightSpeed = 670616629;
lightSpeed /= 10;
// total travel time
double total = 0;
/* your code here */
}
}
```
**Example Output:**
```java
Travel time to ...
Mercury 0 hours
Venus: 0 hours
Mars: 0 hours
Jupiter: 5 hours
Saturn:11 hours
Total travel time:16.0
Travel time to ...
Mercury: 0.8495784968765016 hours
Venus: 0.38359870949813324 hours
Mars: 0.7258725410056196 hours
Jupiter: 5.825604352006665 hours
Saturn: 11.813728535388819 hours
Total travel time:19.59838263477574
```
*Your ouput should look like the example above. I am not asking you to find the **average** for this assignment.*
You will write a widening algorithm with new variables to show the travel time to and from all planets. You will write a casting version without new variables to show the travel times. The one rule is that you must use the provided code and not change any provided data types.
Sample Output
```java
public class FivePlanetTravel
{
public static void main(String[] args)
{
// theplanets.org average distance from earth to the planets
int mercury = 56974146;
int venus = 25724767;
int mars = 48678219;
int jupiter = 390674710;
int saturn = 792248270;
// speed of light and our speed
int lightSpeed = 670616629;
lightSpeed /= 10;
// total travel time
double total = 0;
// number of planets to visit
int numPlanets = 5;
// Auto-widen version
System.out.println("Travel time to ...");
double time = mercury;
time /= lightSpeed;
total += time;
System.out.println(" Mercury " + time + " hours");
time = venus;
time /= lightSpeed;
total += time;
System.out.println(" Venus: " + time + " hours");
time = mars;
time /= lightSpeed;
total += time;
System.out.println(" Mars: " + time + " hours");
time = jupiter;
time /= lightSpeed;
total += time;
System.out.println(" Jupiter: " + time + " hours");
time = saturn;
time /= lightSpeed;
total += time;
System.out.println(" Saturn:" + time + " hours");
System.out.println("Total travel time:" + total);
System.out.println();
// Manually cast version
total = 0;
System.out.println("Travel time to ...");
System.out.println(" Mercury: " + mercury / (double) lightSpeed + " hours");
total += mercury / (double) lightSpeed;
System.out.println(" Venus: " + venus / (double) lightSpeed + " hours");
total += venus / (double) lightSpeed;
System.out.println(" Mars: " + mars / (double) lightSpeed + " hours");
total += mars / (double) lightSpeed;
System.out.println(" Jupiter: " + jupiter / (double) lightSpeed + " hours");
total += jupiter / (double) lightSpeed;
System.out.println(" Saturn: " + saturn / (double) lightSpeed + " hours");
total += saturn / (double) lightSpeed;
System.out.println("Total travel time:" + total);
}
}
```
### Numbers Riddle Project
- [ ] Apply coding concepts you have learned up to this point.
- [ ] Apply a programming development process to create your first project.
- [ ] Capture your project and communicate how each part of your program works.
- [ ] I will demonstrate my understanding of a Unit 1 by writing a program
In this project, you will write a program that incorporates the Java™ concepts you have learned throughout this unit.
Your program will include:
- [ ] Use of the [camelCase](https://betterprogramming.pub/string-case-styles-camel-pascal-snake-and-kebab-case-981407998841) naming convention
- [ ] Both multiline and inline comments -- **see below**
- [ ] The print and printtln methods
- [ ] Variables (appropriately named)
- [ ] Arithmetic expressions
- [ ] The compound assignment operator
- [ ] Conversion between int and double data types
**The Numbers Riddle**
Choose any integer, double it, add six, divide it in half, and subtract the number you started with. The answer is always three!
**Example:**
```java
x = 3 // choose an integer
x = 3 * 2 // double it
x = 6 + 6 // add six
x = 12 / 2 // divide it in half
x = 6 - 3 // subtract the number you started with
x = 3 // answer is always three!
```
**Important:** No shortcuts! You know the answer to the riddle will always be three, but your program is being used to test and validate the riddle. You should print the result of all calculations, not just “3.”
**Requirements of this project**
Your program should allow any number to be stored in a variable and printed out. Then, the program should output its double, output six added to it, output that number divided in half, and, finally, output that number subtracted by the original number. In this way, the program displays each calculation, and of course, the final result of three.
Looking back at previous programs and the requirements stated above, you know you will need:
- [ ] a class definition header that matches the file name
- [ ] the main method of the program
- [ ] a variable defined as the “starting number you choose”
- [ ] You may use a `scanner` class
- [ ] six variables representing integer and double data types and positive, negative, and zero values
- [ ] an algorithm(s) that processes the chosen number
- [ ] a print statement displaying the number you chose
- [ ] a print statement displaying each calculation and the final result
- [ ] comments in your code
- [ ] use of camelCase when appropriate
**Test Cases**
Create variables and choose numbers that match the following six number types to verify your program works for each.
- [ ] Positive Integer
- [ ] Negative Integer
- [ ] Zero
- [ ] One
- [ ] Positive Double
- [ ] Negative Double
**Document and Present**
Comments Requirement
```java
/*=============================================================================
| Assignment: Project 1.6 Numbers Riddle
| Author: [Your First and Last Name (Your E-mail Address)]
|
| Course Name: AP Computer Science A
| Instructor: Mr. Jonathan Virak
| Due Date: [Due Date and Time]
|
| Description: [Describe the program's goal, IN DETAIL.]
|
| Language: [java]
|
| Deficiencies: [If you know of any problems with the code, provide
| details here, otherwise clearly state that you know
| of no unsatisfied requirements and no logic errors.]
*===========================================================================*/
```